mirror of
https://github.com/elastic/kibana.git
synced 2025-04-24 17:59:23 -04:00
[Lens] Show/hide heatmap ticks (#153425)
## Summary Closes https://github.com/elastic/kibana/issues/111880 It adds an extra setting on heatmap axis toolbar settings to hide the tick labels (the same setting also exists on xy charts). A new shared component was created and reused for the XY charts too. 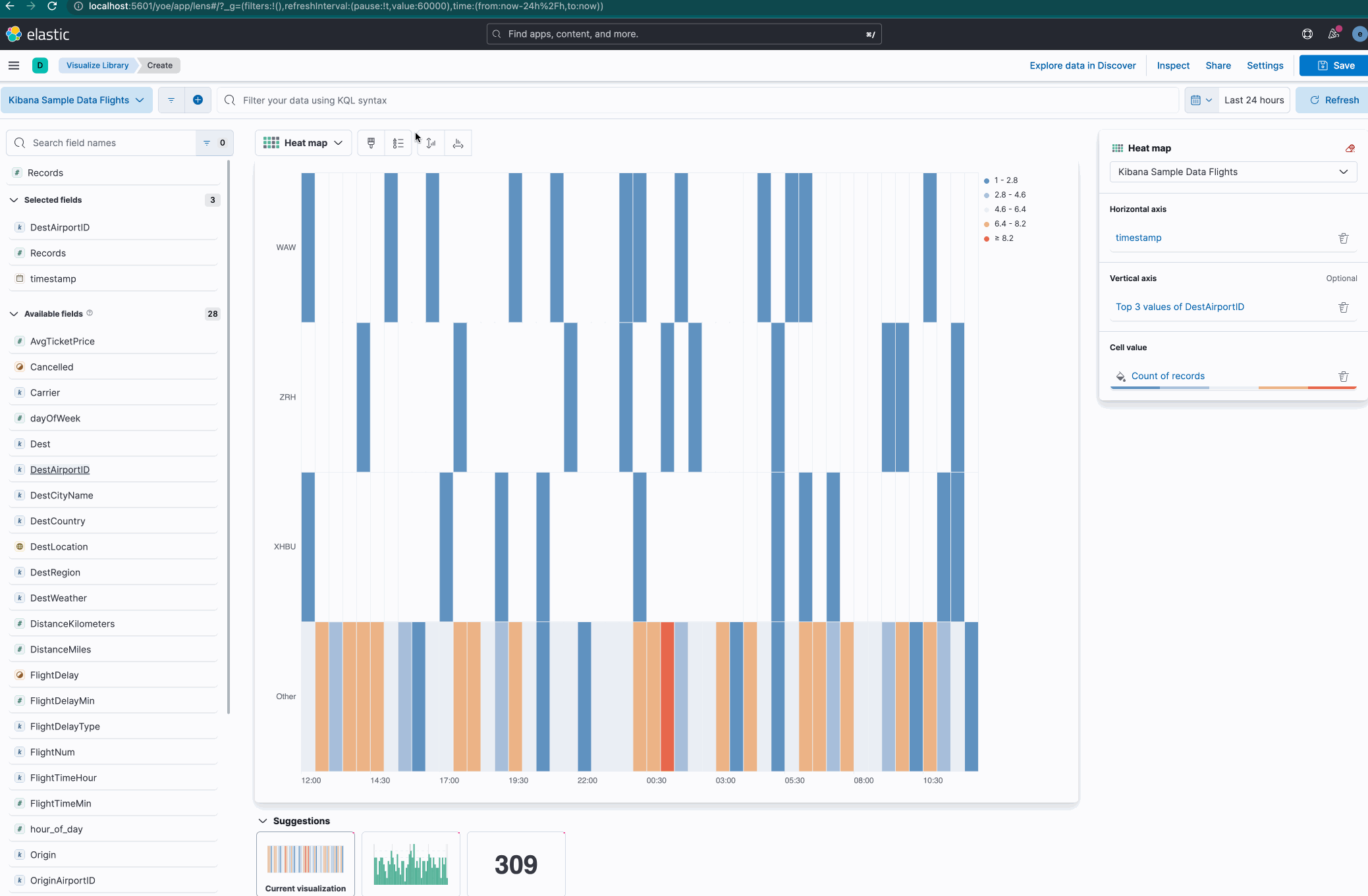 ### Checklist Delete any items that are not applicable to this PR. - [x] Any text added follows [EUI's writing guidelines](https://elastic.github.io/eui/#/guidelines/writing), uses sentence case text and includes [i18n support](https://github.com/elastic/kibana/blob/main/packages/kbn-i18n/README.md) - [x] [Unit or functional tests](https://www.elastic.co/guide/en/kibana/master/development-tests.html) were updated or added to match the most common scenarios - [x] Any UI touched in this PR is usable by keyboard only (learn more about [keyboard accessibility](https://webaim.org/techniques/keyboard/)) - [x] Any UI touched in this PR does not create any new axe failures (run axe in browser: [FF](https://addons.mozilla.org/en-US/firefox/addon/axe-devtools/), [Chrome](https://chrome.google.com/webstore/detail/axe-web-accessibility-tes/lhdoppojpmngadmnindnejefpokejbdd?hl=en-US)) - [x] This renders correctly on smaller devices using a responsive layout. (You can test this [in your browser](https://www.browserstack.com/guide/responsive-testing-on-local-server)) - [x] This was checked for [cross-browser compatibility](https://www.elastic.co/support/matrix#matrix_browsers)
This commit is contained in:
parent
365dd2b931
commit
c908fcdc8e
9 changed files with 172 additions and 24 deletions
|
@ -0,0 +1,61 @@
|
|||
/*
|
||||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
|
||||
* or more contributor license agreements. Licensed under the Elastic License
|
||||
* 2.0; you may not use this file except in compliance with the Elastic License
|
||||
* 2.0.
|
||||
*/
|
||||
|
||||
import React from 'react';
|
||||
import { act } from 'react-dom/test-utils';
|
||||
import { mountWithIntl as mount } from '@kbn/test-jest-helpers';
|
||||
import { EuiSwitch, EuiSwitchEvent } from '@elastic/eui';
|
||||
import { AxisTicksSettings, AxisTicksSettingsProps } from './axis_ticks_settings';
|
||||
|
||||
jest.mock('lodash', () => {
|
||||
const original = jest.requireActual('lodash');
|
||||
|
||||
return {
|
||||
...original,
|
||||
debounce: (fn: unknown) => fn,
|
||||
};
|
||||
});
|
||||
|
||||
describe('Axes Ticks settings', () => {
|
||||
let props: AxisTicksSettingsProps;
|
||||
beforeEach(() => {
|
||||
props = {
|
||||
isAxisLabelVisible: true,
|
||||
axis: 'x',
|
||||
updateTicksVisibilityState: jest.fn(),
|
||||
};
|
||||
});
|
||||
it('should show the ticks switch as on', () => {
|
||||
const component = mount(<AxisTicksSettings {...props} />);
|
||||
expect(
|
||||
component.find('[data-test-subj="lnsshowxAxisTickLabels"]').first().prop('checked')
|
||||
).toBe(true);
|
||||
});
|
||||
|
||||
it('should show the ticks switch as off is the isAxisLabelVisible is set to false', () => {
|
||||
const component = mount(<AxisTicksSettings {...props} isAxisLabelVisible={false} />);
|
||||
expect(
|
||||
component.find('[data-test-subj="lnsshowxAxisTickLabels"]').first().prop('checked')
|
||||
).toBe(false);
|
||||
});
|
||||
|
||||
it('should call the updateTicksVisibilityState when changing the switch status', () => {
|
||||
const updateTicksVisibilityStateSpy = jest.fn();
|
||||
const component = mount(
|
||||
<AxisTicksSettings {...props} updateTicksVisibilityState={updateTicksVisibilityStateSpy} />
|
||||
);
|
||||
|
||||
// switch mode
|
||||
act(() => {
|
||||
component.find(EuiSwitch).first().prop('onChange')({
|
||||
target: { checked: false },
|
||||
} as EuiSwitchEvent);
|
||||
});
|
||||
|
||||
expect(updateTicksVisibilityStateSpy.mock.calls.length).toBe(1);
|
||||
});
|
||||
});
|
|
@ -0,0 +1,62 @@
|
|||
/*
|
||||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one
|
||||
* or more contributor license agreements. Licensed under the Elastic License
|
||||
* 2.0; you may not use this file except in compliance with the Elastic License
|
||||
* 2.0.
|
||||
*/
|
||||
|
||||
import React, { useCallback } from 'react';
|
||||
import { EuiSwitch, EuiFormRow } from '@elastic/eui';
|
||||
import { i18n } from '@kbn/i18n';
|
||||
import type { AxesSettingsConfig } from '../../../visualizations/xy/types';
|
||||
|
||||
type AxesSettingsConfigKeys = keyof AxesSettingsConfig;
|
||||
|
||||
export interface AxisTicksSettingsProps {
|
||||
/**
|
||||
* Determines the axis
|
||||
*/
|
||||
axis: AxesSettingsConfigKeys;
|
||||
/**
|
||||
* Callback to axis ticks status change
|
||||
*/
|
||||
updateTicksVisibilityState: (visible: boolean, axis: AxesSettingsConfigKeys) => void;
|
||||
/**
|
||||
* Determines if the axis tick labels are visible
|
||||
*/
|
||||
isAxisLabelVisible: boolean;
|
||||
}
|
||||
|
||||
export const AxisTicksSettings: React.FunctionComponent<AxisTicksSettingsProps> = ({
|
||||
axis,
|
||||
isAxisLabelVisible,
|
||||
updateTicksVisibilityState,
|
||||
}) => {
|
||||
const onTicksStatusChange = useCallback(
|
||||
(visible) => updateTicksVisibilityState(visible, axis),
|
||||
[axis, updateTicksVisibilityState]
|
||||
);
|
||||
|
||||
return (
|
||||
<>
|
||||
<EuiFormRow
|
||||
display="columnCompressedSwitch"
|
||||
label={i18n.translate('xpack.lens.shared.tickLabels', {
|
||||
defaultMessage: 'Tick labels',
|
||||
})}
|
||||
fullWidth
|
||||
>
|
||||
<EuiSwitch
|
||||
compressed
|
||||
data-test-subj={`lnsshow${axis}AxisTickLabels`}
|
||||
label={i18n.translate('xpack.lens.shared.tickLabels', {
|
||||
defaultMessage: 'Tick labels',
|
||||
})}
|
||||
onChange={() => onTicksStatusChange(!isAxisLabelVisible)}
|
||||
checked={isAxisLabelVisible}
|
||||
showLabel={false}
|
||||
/>
|
||||
</EuiFormRow>
|
||||
</>
|
||||
);
|
||||
};
|
|
@ -36,6 +36,7 @@ export { LegendActionPopover } from './legend/action/legend_action_popover';
|
|||
export { NameInput } from './name_input';
|
||||
export { ValueLabelsSettings } from './value_labels_settings';
|
||||
export { AxisTitleSettings } from './axis/title/axis_title_settings';
|
||||
export { AxisTicksSettings } from './axis/ticks/axis_ticks_settings';
|
||||
export { DimensionEditorSection } from './dimension_section';
|
||||
export { FilterQueryInput } from './filter_query_input';
|
||||
export * from './static_header';
|
||||
|
|
|
@ -18,6 +18,7 @@ import {
|
|||
ValueLabelsSettings,
|
||||
AxisTitleSettings,
|
||||
TooltipWrapper,
|
||||
AxisTicksSettings,
|
||||
} from '../../shared_components';
|
||||
import type { HeatmapVisualizationState } from './types';
|
||||
import { getDefaultVisualValuesForLayer } from '../../shared_components/datasource_default_values';
|
||||
|
@ -165,6 +166,19 @@ export const HeatmapToolbar = memo(
|
|||
}}
|
||||
isAxisTitleVisible={state?.gridConfig.isYAxisTitleVisible}
|
||||
/>
|
||||
<AxisTicksSettings
|
||||
axis="yLeft"
|
||||
updateTicksVisibilityState={(visible) => {
|
||||
setState({
|
||||
...state,
|
||||
gridConfig: {
|
||||
...state.gridConfig,
|
||||
isYAxisLabelVisible: visible,
|
||||
},
|
||||
});
|
||||
}}
|
||||
isAxisLabelVisible={state?.gridConfig.isYAxisLabelVisible}
|
||||
/>
|
||||
</ToolbarPopover>
|
||||
</TooltipWrapper>
|
||||
|
||||
|
@ -198,6 +212,19 @@ export const HeatmapToolbar = memo(
|
|||
}
|
||||
isAxisTitleVisible={state?.gridConfig.isXAxisTitleVisible}
|
||||
/>
|
||||
<AxisTicksSettings
|
||||
axis="x"
|
||||
updateTicksVisibilityState={(visible) => {
|
||||
setState({
|
||||
...state,
|
||||
gridConfig: {
|
||||
...state.gridConfig,
|
||||
isXAxisLabelVisible: visible,
|
||||
},
|
||||
});
|
||||
}}
|
||||
isAxisLabelVisible={state?.gridConfig.isXAxisLabelVisible}
|
||||
/>
|
||||
</ToolbarPopover>
|
||||
</TooltipWrapper>
|
||||
</EuiFlexGroup>
|
||||
|
|
|
@ -8,7 +8,7 @@
|
|||
import React from 'react';
|
||||
import { shallowWithIntl as shallow } from '@kbn/test-jest-helpers';
|
||||
import { AxisSettingsPopover, AxisSettingsPopoverProps } from './axis_settings_popover';
|
||||
import { ToolbarPopover } from '../../../shared_components';
|
||||
import { ToolbarPopover, AxisTicksSettings } from '../../../shared_components';
|
||||
import { LayerTypes } from '@kbn/expression-xy-plugin/public';
|
||||
import { ShallowWrapper } from 'enzyme';
|
||||
|
||||
|
@ -67,13 +67,23 @@ describe('Axes Settings', () => {
|
|||
});
|
||||
|
||||
it('has the tickLabels switch on by default', () => {
|
||||
const component = shallow(<AxisSettingsPopover {...props} />);
|
||||
const component = shallow(
|
||||
<AxisTicksSettings
|
||||
axis={props.axis}
|
||||
isAxisLabelVisible={props.areTickLabelsVisible}
|
||||
updateTicksVisibilityState={jest.fn()}
|
||||
/>
|
||||
);
|
||||
expect(component.find('[data-test-subj="lnsshowxAxisTickLabels"]').prop('checked')).toBe(true);
|
||||
});
|
||||
|
||||
it('has the tickLabels switch off when tickLabelsVisibilitySettings for this axes are false', () => {
|
||||
const component = shallow(
|
||||
<AxisSettingsPopover {...props} axis="yLeft" areTickLabelsVisible={false} />
|
||||
<AxisTicksSettings
|
||||
axis="yLeft"
|
||||
isAxisLabelVisible={false}
|
||||
updateTicksVisibilityState={jest.fn()}
|
||||
/>
|
||||
);
|
||||
expect(component.find('[data-test-subj="lnsshowyLeftAxisTickLabels"]').prop('checked')).toBe(
|
||||
false
|
||||
|
|
|
@ -23,6 +23,7 @@ import {
|
|||
useDebouncedValue,
|
||||
AxisTitleSettings,
|
||||
AxisBoundsControl,
|
||||
AxisTicksSettings,
|
||||
} from '../../../shared_components';
|
||||
import { XYLayerConfig, AxesSettingsConfig } from '../types';
|
||||
import { validateExtent } from '../axes_configuration';
|
||||
|
@ -292,24 +293,13 @@ export const AxisSettingsPopover: React.FunctionComponent<AxisSettingsPopoverPro
|
|||
/>
|
||||
</EuiFormRow>
|
||||
|
||||
<EuiFormRow
|
||||
display="columnCompressedSwitch"
|
||||
label={i18n.translate('xpack.lens.xyChart.tickLabels', {
|
||||
defaultMessage: 'Tick labels',
|
||||
})}
|
||||
fullWidth
|
||||
>
|
||||
<EuiSwitch
|
||||
compressed
|
||||
data-test-subj={`lnsshow${axis}AxisTickLabels`}
|
||||
label={i18n.translate('xpack.lens.xyChart.tickLabels', {
|
||||
defaultMessage: 'Tick labels',
|
||||
})}
|
||||
onChange={() => toggleTickLabelsVisibility(axis)}
|
||||
checked={areTickLabelsVisible}
|
||||
showLabel={false}
|
||||
/>
|
||||
</EuiFormRow>
|
||||
<AxisTicksSettings
|
||||
axis={axis}
|
||||
updateTicksVisibilityState={(visible) => {
|
||||
toggleTickLabelsVisibility(axis);
|
||||
}}
|
||||
isAxisLabelVisible={areTickLabelsVisible}
|
||||
/>
|
||||
{!useMultilayerTimeAxis && areTickLabelsVisible && (
|
||||
<EuiFormRow
|
||||
display="columnCompressed"
|
||||
|
|
|
@ -20297,7 +20297,6 @@
|
|||
"xpack.lens.xyChart.showCurrenTimeMarker": "Afficher le repère de temps actuel",
|
||||
"xpack.lens.xyChart.showEnzones": "Afficher les marqueurs de données partielles",
|
||||
"xpack.lens.xyChart.splitSeries": "Répartition",
|
||||
"xpack.lens.xyChart.tickLabels": "Étiquettes de graduation",
|
||||
"xpack.lens.xyChart.tooltip": "Infobulle",
|
||||
"xpack.lens.xyChart.topAxisDisabledHelpText": "Ce paramètre s'applique uniquement lorsque l'axe du haut est activé.",
|
||||
"xpack.lens.xyChart.topAxisLabel": "Axe du haut",
|
||||
|
|
|
@ -20297,7 +20297,6 @@
|
|||
"xpack.lens.xyChart.showCurrenTimeMarker": "現在時刻マーカーを表示",
|
||||
"xpack.lens.xyChart.showEnzones": "部分データマーカーを表示",
|
||||
"xpack.lens.xyChart.splitSeries": "内訳",
|
||||
"xpack.lens.xyChart.tickLabels": "目盛ラベル",
|
||||
"xpack.lens.xyChart.tooltip": "ツールチップ",
|
||||
"xpack.lens.xyChart.topAxisDisabledHelpText": "この設定は、上の軸が有効であるときにのみ適用されます。",
|
||||
"xpack.lens.xyChart.topAxisLabel": "上の軸",
|
||||
|
|
|
@ -20297,7 +20297,6 @@
|
|||
"xpack.lens.xyChart.showCurrenTimeMarker": "显示当前时间标记",
|
||||
"xpack.lens.xyChart.showEnzones": "显示部分数据标记",
|
||||
"xpack.lens.xyChart.splitSeries": "细目",
|
||||
"xpack.lens.xyChart.tickLabels": "刻度标签",
|
||||
"xpack.lens.xyChart.tooltip": "工具提示",
|
||||
"xpack.lens.xyChart.topAxisDisabledHelpText": "此设置仅在启用顶轴时应用。",
|
||||
"xpack.lens.xyChart.topAxisLabel": "顶轴",
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue